닉네임으로 해당 유저의 정보 가져오기
Controller
@PostMapping("/riot/summonerByName")
public Summoner callSummonerByName(@RequestParam String summonerName){
summonerName = summonerName.replaceAll(" ","%20");
Summoner apiResult = riotService.callRiotAPISummonerByName(summonerName);
return apiResult;
}
닉네임 입력시 해당 유저의 정보를 끌어옴 + 현재는 profileIconId는 사용하지 않을 것 같아서 받아오지 않는데, 나중에 사용할거면 추가할 예정
Service
public Summoner callRiotAPISummonerByName(String nickname){
Optional<Summoner> find_summoner = riotRepository.findByNickname(nickname);
if(find_summoner.isPresent()){
//log.info("이미 db에 존재");
return find_summoner.get();
}
SummonerDTO result;
String serverUrl = "https://kr.api.riotgames.com";
try {
CloseableHttpClient client = HttpClientBuilder.create().build();
HttpGet request = new HttpGet(serverUrl + "/lol/summoner/v4/summoners/by-name/" + nickname + "?api_key=" + mykey);
HttpResponse response = (HttpResponse) client.execute(request);
if(response.getStatusLine().getStatusCode() != 200){
// 오류
return null;
}
HttpEntity entity = response.getEntity();
result = objectMapper.readValue(entity.getContent(), SummonerDTO.class);
Summoner summoner = result.toEntity();
riotRepository.save(summoner);
return summoner;
} catch (IOException e){
e.printStackTrace();
return null;
}
}
라이엇에서 제공하는 api를 SummonerDTO형식으로 받아오고, SummonerDTO의 toEntity를 사용해 Summoner db에 저장할 때는 내가 원하는 컬럼만 저장한다.
테스트 결과 DB:
puuid로 최근 경기 id 가져오기
Controller
@PostMapping("/riot/match")
public String[] getMatchInfo(@RequestParam String puuid){
return riotService.getMatchInfo(puuid);
}
위에서 얻은 puuid를 입력하면 최근 경기 5판의 id를 얻을 수 있다.
Service
public String[] getMatchInfo(String puuid){
try {
CloseableHttpClient client = HttpClientBuilder.create().build();
HttpGet request = new HttpGet("https://asia.api.riotgames.com/lol/match/v5/matches/by-puuid/" + puuid +"/ids?type=ranked&start=0&count=5&api_key=" + mykey);
HttpResponse response = (HttpResponse) client.execute(request);
HttpEntity entity = response.getEntity();
String matchIds = EntityUtils.toString(entity);
String substring = matchIds.substring(2, matchIds.length() - 2); // 양 옆의 [", "] 없애기
System.out.println(substring);
String[] matchIdArray = substring.split("\",\"");
// for (String s : matchIdArray) {
// System.out.println(s);
// }
return matchIdArray;
} catch (IOException e){
e.printStackTrace();
return null;
}
}
우선은 경기의 id를 배열형태로 출력만 하는데, 만약 그 경기들의 정보도 같이 리턴하고싶으면 다음에 for문 안에 matchDetailInfo를 넣어준다.
테스트
경기 id로 경기 정보 받기
Riot API - ResponseBody
{
"metadata": {
"dataVersion": "2",
"matchId": "KR_6654615721",
"participants": [
"N3IdH3P5AFpt2nJ5DRGkw0gJF2IMrdH8YiuyTEwxwx3ctJyIcbrUNoGdbP0oAtSudLGi--qmtkJLrA",
"4R_EE52N--p5f58N0OroN_dRoHBPHHSmLewgBK7f1Hyxn1_U6c4sXtPSFlMoebwRvRV4BEIV-F7Kgg",
"_lY3koy_l_a00gEaSjh98ROMkzZGC4CX-eiF6n5YxtL-bnKjjkUbB_9wJ6zMFeugm9DbfeZOc9j-Vg",
"VC-U-mnqEqVE-p0_ULnIB0UrOLkwZ9JAN71Ax1swx6ILkkJ0e9XIc6h2TNa2M0OIVDCiOO1wn1-kyw",
"iwA0Qygpv_Dscw5Yu9uozKGGgus-WiUy9b78rT1lEI7KfmtpReR5Xvl4KerFMUYxhe6BnV8oMUGnYA",
"oiE5B9H__DHwJtZfMj3CExHcxl0-zQFAUs0NffAEOQsxsk-cdZEEAkyuq_ix9VFNHYbGcNgxlwoIEA",
"IcDNiZcFgltqF9n54dmEJLSQht7QrjHr2x9xAM8R9vh9Rw-ocuQqNME3qhYR0UitbnP0Ngag9J5tgw",
"6LVmq4ZauB09Bkc-rpbb0LWaEdRe64TWEZEl5ftb-tZE4jfzs2gZfpkKjJHOQ51F-HCmBbti38aNrA",
"vE8RX81evkZycWEgvegcMxbTNs8Noza--yEL92ZLJCTaEtgAdhBoG0ycudIPt6HYih_rTPnNlK0Xog",
"PHRUL4IciG8SLtNf_EAWL3s5SHSkymUovsA1Z4-q4jaFwvydTMx0rG_JhFe0FvSdUrLfIYllXKoldw"
]
},
"info": {
"gameCreation": 1692124317188,
"gameDuration": 2411,
"gameEndTimestamp": 1692126744397,
"gameId": 6654615721,
"gameMode": "CLASSIC",
"gameName": "teambuilder-match-6654615721",
"gameStartTimestamp": 1692124333440,
"gameType": "MATCHED_GAME",
"gameVersion": "13.15.524.1760",
"mapId": 11,
"participants": [
{
"allInPings": 0,
"assistMePings": 0,
"assists": 17,
"baitPings": 0,
"baronKills": 0,
몇 천줄이 되는 게임 정보 중 내가 원하는 정보만 뽑아 올 예정
Controller
@PostMapping("/riot/game")
public JsonObject getGameInfo(@RequestParam String matchId) throws IOException, ParseException {
return riotService.matchDetailInfo(matchId);
}
해당 경기의 정보를 리턴해주는 컨트롤러
Service
public JsonObject matchDetailInfo(String matchId) throws IOException, ParseException {
CloseableHttpClient client = HttpClientBuilder.create().build();
HttpGet request = new HttpGet("https://asia.api.riotgames.com/lol/match/v5/matches/" + matchId +"?api_key=" + mykey);
HttpResponse response = (HttpResponse) client.execute(request);
HttpEntity entity = response.getEntity();
String matchInfo = EntityUtils.toString(entity);
JSONParser parser = new JSONParser();
Object obj = parser.parse(matchInfo);
JSONObject jsonObj = (JSONObject) obj;
// JSONObject jso = (JSONObject) jsonObj.get("metadata");
JSONObject info = (JSONObject) jsonObj.get("info");
JSONArray participants = (JSONArray) info.get("participants");
JsonObject jsonobject = new JsonObject();
jsonobject.addProperty("gameMode", String.valueOf(info.get("gameMode")));
jsonobject.addProperty("gameDuration", String.valueOf(info.get("gameDuration")));
JsonArray infoArray = new JsonArray();
for (int i=0; i< participants.size(); i++) {
JSONObject participant = (JSONObject) participants.get(i);
JsonObject userInfo = new JsonObject();
userInfo.addProperty("summonerName", (String) participant.get("summonerName"));
userInfo.addProperty("championName", (String) participant.get("championName"));
userInfo.addProperty("champLevel",String.valueOf(participant.get("champLevel")));
userInfo.addProperty("individualPosition", (String) participant.get("individualPosition"));
userInfo.addProperty("kills",String.valueOf(participant.get("kills")));
userInfo.addProperty("deaths",String.valueOf(participant.get("deaths")));
userInfo.addProperty("assists",String.valueOf(participant.get("assists")));
userInfo.addProperty("totalDamageDealtToChampions",String.valueOf(participant.get("totalDamageDealtToChampions")));
userInfo.addProperty("totalDamageTaken",String.valueOf(participant.get("totalDamageTaken")));
userInfo.addProperty("goldEarned",String.valueOf(participant.get("goldEarned")));
userInfo.addProperty("item0",String.valueOf(participant.get("item0")));
userInfo.addProperty("item1",String.valueOf(participant.get("item1")));
userInfo.addProperty("item2",String.valueOf(participant.get("item2")));
userInfo.addProperty("item3",String.valueOf(participant.get("item3")));
userInfo.addProperty("item4",String.valueOf(participant.get("item4")));
userInfo.addProperty("item5",String.valueOf(participant.get("item5")));
userInfo.addProperty("item6",String.valueOf(participant.get("item6")));
userInfo.addProperty("wardsPlaced",String.valueOf(participant.get("wardsPlaced")));
userInfo.addProperty("wardsKilled",String.valueOf(participant.get("wardsKilled")));
infoArray.add(userInfo);
jsonobject.add("users", infoArray);
}
return jsonobject;
}
api를 통해 받은 정보를 Json형태로 변경하는 데 오래걸렸다.
VO를 만들어서 받기에는 정보가 너무 많아서 EntityUtils를 통해 String형으로 받고 그걸 Object로 변경해서 그걸 또 JsonObject로 받는다.. (더 편한 방법이 있을 것 같은데 나는 못찾겠다..) 찾아보니 Map형식으로 받는 것도 가능할 것같은데 다음에 사용해보자..
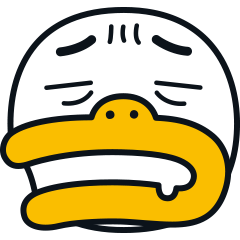
Json 형식으로 출력하는 건 이 블로그에서 도움을 받았다.
그래서.. Json 형식으로 출력을 하려는데 에러 발생 ㅠㅠ
2023-08-16T17:30:33.341+09:00 WARN 92510 --- [nio-8080-exec-2] .w.s.m.s.DefaultHandlerExceptionResolver : Resolved [org.springframework.http.converter.HttpMessageNotWritableException: Could not write JSON: JsonObject]
application.yml
spring:
mvc:
converters:
preferred-json-mapper: gson
추가해주니 정상적으로 작동했다 ㅎㅎ
테스트:
스웨거 정리 완료!
'개발' 카테고리의 다른 글
[Mysql] orphanRemoval vs CascadeType.REMOVE (0) | 2023.08.28 |
---|---|
[스프링부트] Querydsl 쿼리문 작성 (닉네임 공백, 대소문자) (0) | 2023.08.21 |
[SpringBoot] 이메일 인증 구현 (0) | 2023.08.11 |
사이트 디자인 + DB설계(수정 필요) (0) | 2023.08.04 |
[CI/CD] S3 + CodeDeploy (0) | 2023.08.01 |